Snapshot Testing
βοΈ Edit this pageAdding snapshot tests with Jest is a great way to help avoid unintended changes to your app's UI.
By diffing the serialized value of your React tree Jest can show you what changed in your app and allow you to fix it or update the snapshot.
By default snapshots with emotion show generated class names. Adding @emotion/jest allows you to output the actual styles being applied.
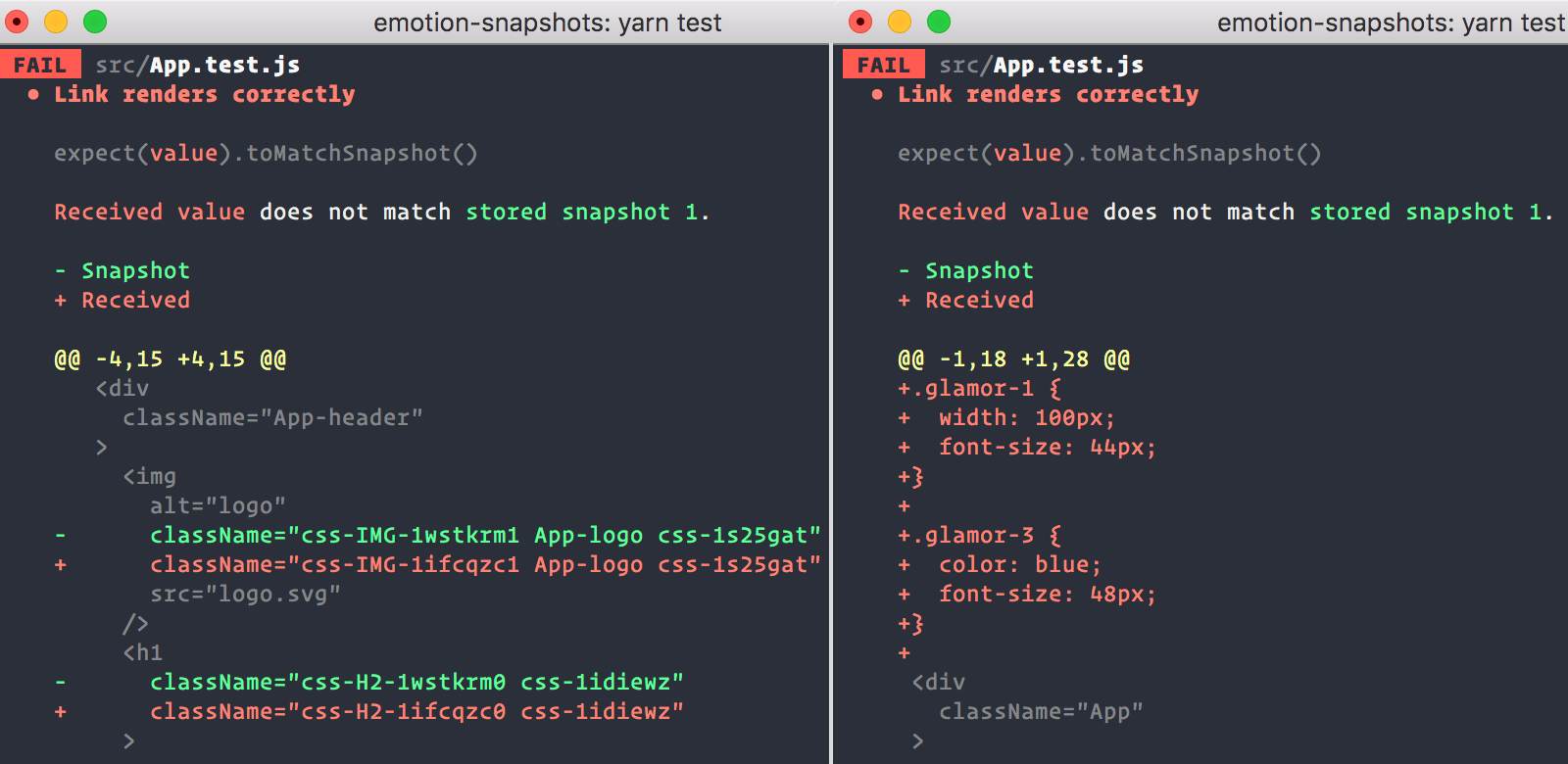
Installation
npm install --save-dev @emotion/jest
Add the "@emotion/jest/serializer"
to the snapshotSerializers
option.
{
"snapshotSerializers": ["@emotion/jest/serializer"]
}
Or use expect.addSnapshotSerializer
to add it.
import { createSerializer } from '@emotion/jest'
expect.addSnapshotSerializer(createSerializer())
When using Enzyme, you can add "@emotion/jest/enzyme-serializer"
instead.
{
"snapshotSerializers": ["@emotion/jest/enzyme-serializer"]
}
Or use expect.addSnapshotSerializer
to add it like this:
// also adds the enzyme-to-json serializer
import { createEnzymeSerializer } from '@emotion/jest/enzyme-serializer'
expect.addSnapshotSerializer(createEnzymeSerializer())
Writing a test
Writing a test with @emotion/jest
involves creating a snapshot from the react-test-renderer
or enzyme-to-json
's resulting JSON.
import React from 'react'
import renderer from 'react-test-renderer'
const Button = props => (
<button
css={{
color: 'hotpink'
}}
{...props}
/>
)
test('Button renders correctly', () => {
expect(
renderer.create(<Button>This is hotpink.</Button>).toJSON()
).toMatchSnapshot()
})
It'll create a snapshot that looks like this.
// Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`Button renders correctly 1`] = `
.emotion-0 {
color: hotpink;
}
<div
className="emotion-0"
>
This is hotpink.
</div>
`
When the styles of a component change, the snapshot will fail and you'll be able to update the snapshot or fix the component.